With the help of someone online, I was able to generate 90% complete toolbox label for 3D printing using OpenSCAD. Being able to generate your 3D model in code makes for easy repetition.
$fn = 64;
outline(0.5)
text("Spencer", font = "Serpentine");
module outline(border) {
bridging = 5;
color("black")
linear_extrude(1.5)
offset(delta = border)
offset(delta = -bridging)
offset(delta = bridging)
children();
color("silver")
translate([0, 0, 0.2])
linear_extrude(1.5)
children();
}
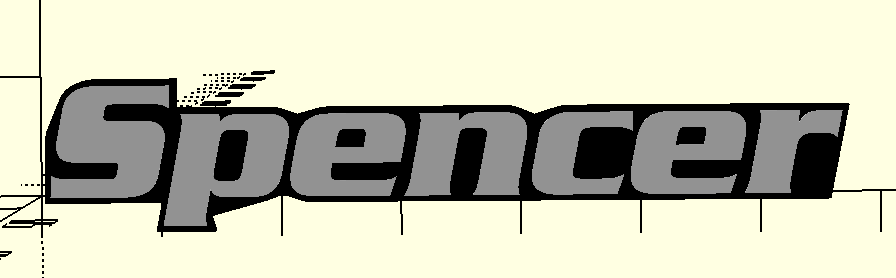
New Approach for 2024 dev version of OpenSCAD
// Only for a new 2024 version of OpenSCAD.
// Find your own Serpentine font.
use <Serpentine.ttf>
fnt = "Serpentine:style=Bold Italic";
txt = "Hoffman";
border = 0.7;
melting_border = 3;
line_offset = 12;
// A list with the x-coornates
// of all the characters.
listx = [ for(i=[0:len(txt)-1]) GetCharX(txt,i) ];
// Print just the text
color("Black")
translate([0,3*line_offset])
text(txt,font=fnt);
// Print squares for each character
translate([0,2*line_offset])
{
color("Orange")
translate([0,0,2])
text(txt,font=fnt);
color("Green")
for(i=[0:len(txt)-1])
translate([listx[i],0])
translate(textmetrics(txt[i],font=fnt).position)
square(textmetrics(txt[i],font=fnt).size);
}
// With delta offset
translate([0,1*line_offset])
{
color("Gold")
translate([0,0,2])
text(txt,font=fnt);
color("Blue")
offset(delta=-(melting_border-border),chamfer=false)
for(i=[0:len(txt)-1])
offset(delta=melting_border,chamfer=false)
translate([listx[i],0])
text(txt[i],font=fnt);
}
// Double offset to fix the the weird
// shape of the 'H' at the upper-right.
translate([0,0*line_offset])
{
color("Silver")
translate([0,0,2])
text(txt,font=fnt);
color("Navy")
{
// Offset 1
offset(delta=-(melting_border-border),chamfer=false)
for(i=[0:len(txt)-1])
offset(delta=melting_border,chamfer=false)
translate([listx[i],0])
text(txt[i],font=fnt);
// Offset 2
for(i=[0:len(txt)-1])
offset(delta=border,chamfer=false)
translate([listx[i],0])
text(txt[i],font=fnt);
}
}
// This function gets the 'x' position
// of a character in the string,
// but the textmetric() parameters must be
// the same as the text() parameters!
function GetCharX(t,i=0) =
i > 0 ?
textmetrics(t[i-1],font=fnt).advance[0] + GetCharX(t,i-1) :
0;